Alright, let’s talk about this “backfire news” thing I messed around with today. It was a bit of a wild ride, honestly.
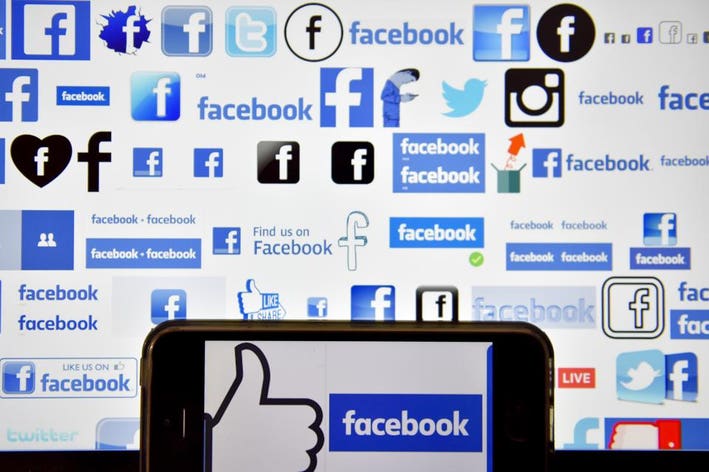
So, it all started when I saw this post mentioning needing some sort of news feed thingy. My brain immediately went to, “Hey, I can probably hack something together!” Famous last words, right?
First thing I did was grab Python. Gotta start somewhere. Then I figured, “Okay, news needs a source.” Duh. I remembered seeing some stuff about RSS feeds, so I went down that rabbit hole. Found a bunch of RSS feeds from different news sites. I just grabbed a few random ones to test with – BBC, CNN, you know, the usual suspects.
Next up was finding a Python library to actually read these RSS feeds. After a bit of googling, I landed on `feedparser`. Seemed simple enough. I installed it with pip – `pip install feedparser`, boom done.
Okay, time to actually write some code. I started with a basic script that just takes an RSS feed URL, parses it, and then prints out the titles of the news articles. Pretty straightforward stuff:
- Import `feedparser`
- Define a function that takes a URL as input
- Use `*()` to parse the feed
- Loop through the `entries` in the parsed feed
- Print the title of each entry
Ran it, and bam! Titles started showing up in my terminal. Felt like a genius for a solid 30 seconds.
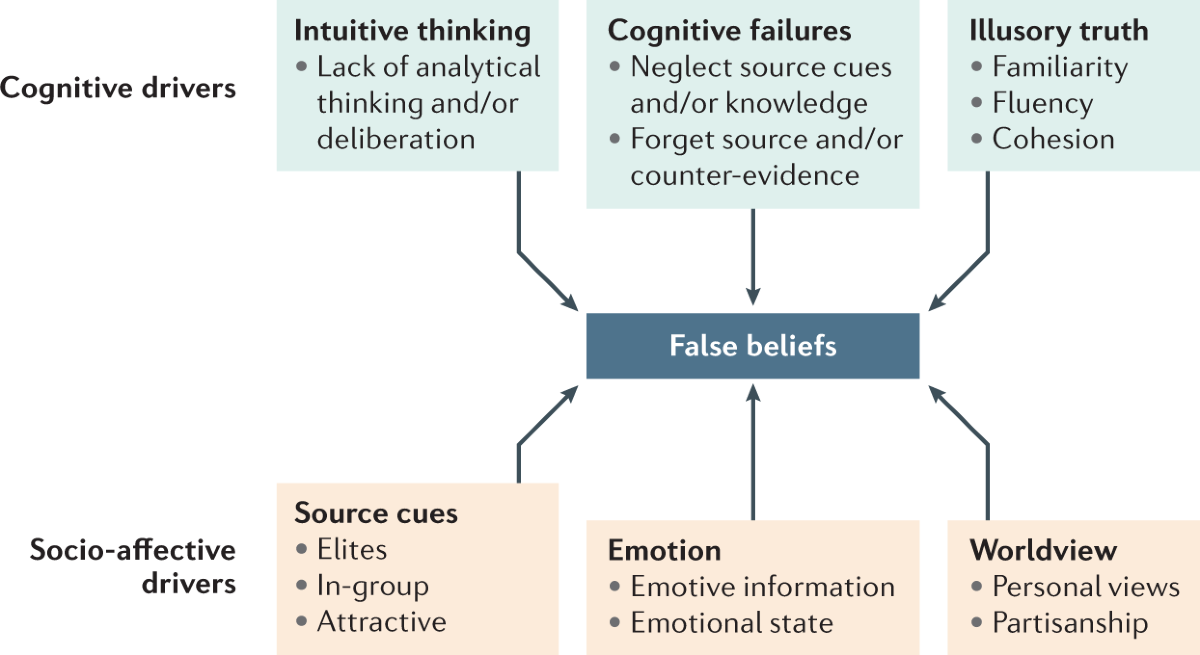
But just spitting out titles wasn’t good enough. I wanted to see the summaries too. So I modified the script to also print the `summary` for each article. Easy peasy.
Now, here’s where things got a little messy. The summaries were full of HTML tags. `
`, ``, all that jazz. Ugly as sin. I needed to strip those out. Found a library called `BeautifulSoup4` to handle that. Installed it with `pip install beautifulsoup4`.
Then I tweaked my code to use BeautifulSoup to clean up the summaries. Basically, I created a BeautifulSoup object from the summary HTML, and then just got the text out of it. Worked like a charm! No more HTML tags.
So, at this point, I had a script that could grab RSS feeds, parse them, and print out the titles and clean summaries. Cool. But I wanted to make it look a little nicer. Just printing stuff to the terminal is boring.
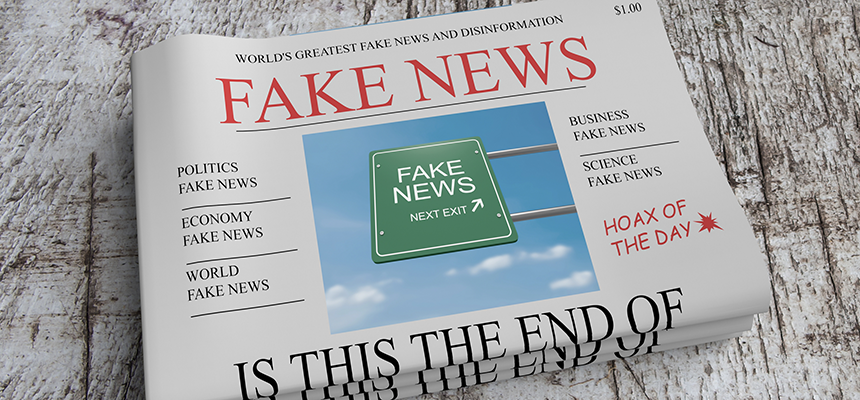
I decided to throw together a quick and dirty web interface using Flask. I know, I know, probably overkill, but I wanted to play around with it. Installed Flask with `pip install Flask`.
Created a simple Flask app with a single route that would:
- Take an RSS feed URL as a parameter
- Use my existing code to parse the feed and get the articles
- Render a simple HTML template to display the articles with their titles and summaries
The HTML template was super basic. Just a loop that iterated through the articles and displayed each one in a `
Fired up the Flask app, pointed my browser to it, and… it worked! I had a rudimentary “news” website displaying articles from the RSS feeds. It was ugly as hell, but it was mine.
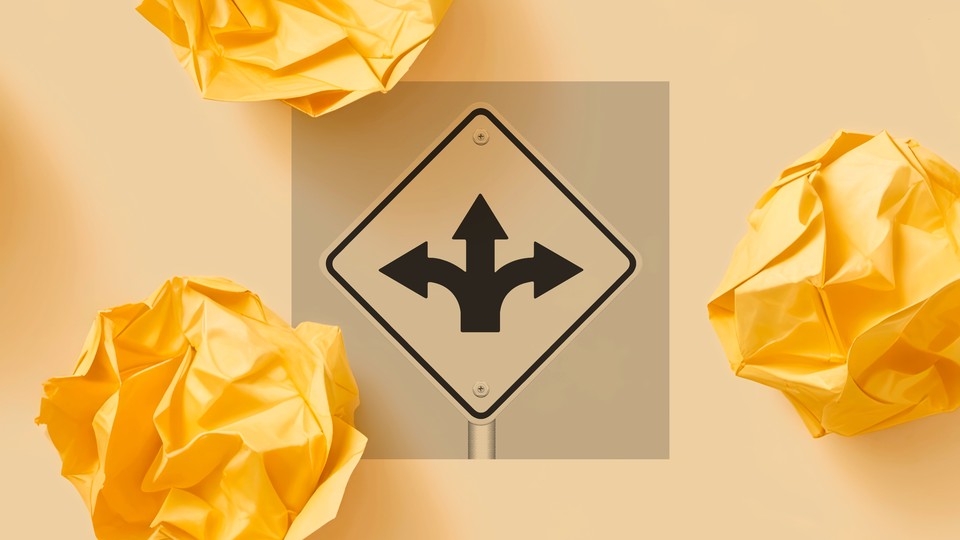
Of course, there are a million things I could do to improve it. Add error handling, make it look prettier, allow users to choose their own RSS feeds, maybe even add some kind of search functionality. But for a quick little project, I’m pretty happy with how it turned out.
It’s all about just jumping in and figuring things out as you go. Don’t be afraid to experiment and make mistakes. That’s how you learn. And don’t worry about making it perfect. Just get something working first, and then iterate on it.
Anyway, that was my “backfire news” adventure. Hope you found it somewhat interesting or at least mildly amusing. Maybe I’ll clean it up and make it into something actually useful someday. Who knows?