Okay, so yesterday I was messing around with this thing called “saltarsela.” Sounds fancy, right? It ain’t. It’s just a way to, like, skip over stuff in a process. I needed it ’cause I was trying to automate this really tedious data cleaning task. Picture this: tons of spreadsheets, all formatted differently, and I gotta pull specific info from each one. Ugh.
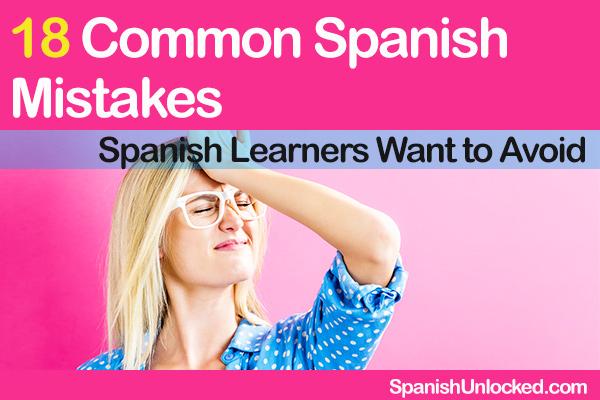
First, I started by mapping out the whole process. I literally grabbed a whiteboard and drew a flowchart. It looked like a kindergartener did it, but hey, it worked. I identified the steps that were consistently failing or just plain unnecessary depending on the spreadsheet. These were the prime candidates for the “saltarsela” treatment.
Then, I dug into the scripting part. I’m using Python, ’cause it’s what I know. I started with a basic loop that iterated through each spreadsheet. The key was to add `if` statements to check for certain conditions. Like, “if this cell is empty, skip to the next sheet.” Or, “if this column contains the word ‘deprecated,’ jump to step X.”
The tricky part was figuring out how to actually “skip.” I tried a few things. `continue` worked for skipping to the next iteration of the loop, but it didn’t help when I needed to jump multiple steps ahead. I also played around with `goto`, but everyone online says that’s a big no-no, and honestly, it made my code look even messier.
What finally worked was breaking the main loop into smaller functions. Each function handled a specific step in the data cleaning process. Then, inside each function, I could use `return` to effectively “jump” to another function. It’s kind of like building a chain of functions, and depending on the data, you can skip links in the chain.
For example:
- Function A: Checks for specific conditions in the sheet.
- If condition 1 is met, return and call Function C.
- If condition 2 is met, return and call Function B.
- Otherwise, execute the default actions within Function A.
This approach made the code more readable and easier to debug. Plus, it allowed me to add more “saltarselas” later on without completely rewriting everything.
After writing the functions, I tested it like crazy. I threw all sorts of messed-up spreadsheets at it, and slowly but surely, I ironed out the bugs. Turns out, dealing with inconsistent data is a never-ending battle, but at least now I have a weapon – the “saltarsela.”
The end result? My data cleaning time went from hours to minutes. Not bad for a day’s work, eh?