Alright, let’s talk about this `tokio test` thing. If you’ve ever wrestled with async Rust, you know testing can feel like another beast entirely. It’s not like your plain old synchronous code where you just call a function and check the result. Oh no, async throws a wrench in that, especially when you’re deep in Tokio-land.
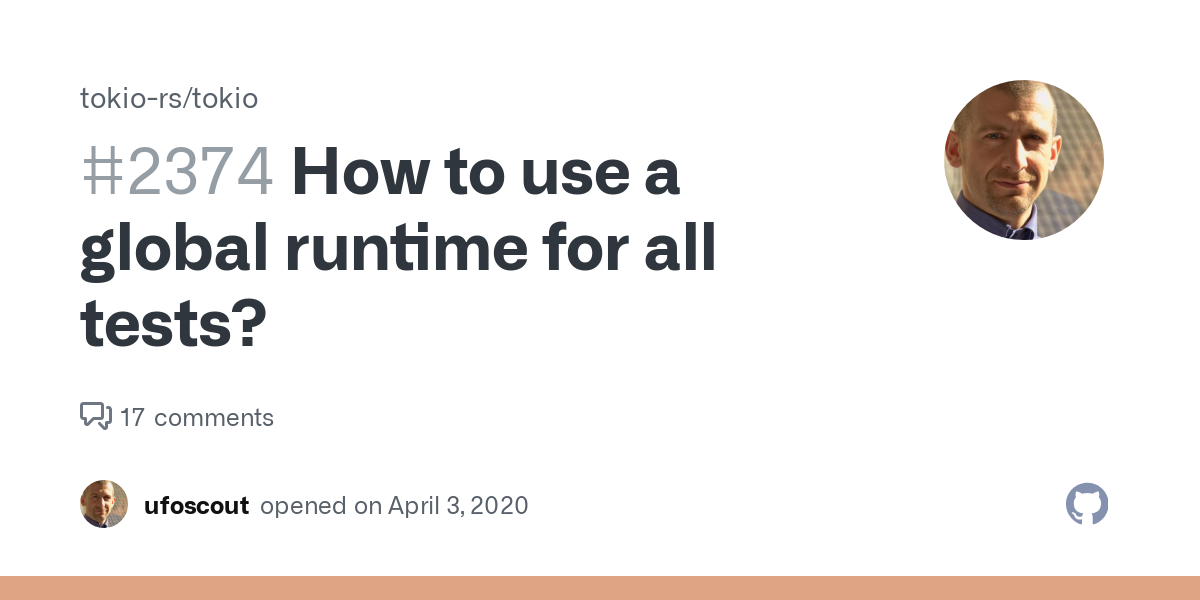
My Early Async Testing Mess
I remember when I first started getting serious with Tokio for a little side project, a network utility kind of thing. Writing the async logic itself was one hurdle, but then came testing. My usual `#[test]` just wasn’t cutting it. I’d write an async test function, and it would complain, or I’d try to `block_on` inside every test, and it just felt clunky and wrong. It was a real pain, honestly. My test suites were getting ugly with all that manual runtime setup for every single async test. I spent more time fiddling with the test harness than writing the actual test logic. It was frustrating, to say the least.
Stumbling Onto the Solution
I think I was complaining to a colleague, or maybe just ranting to myself late one night, about how messy this all was. Then, either through some desperate searching or a lucky find in the Tokio docs, I bumped into the `#[tokio::test]` attribute. At first, I was skeptical. Just slap this on top of my async test function and it all works? Seemed too good to be true, given my previous struggles.
So, I decided to give it a shot. I took one of my simpler async functions, something that maybe did a small `await` for a timer and returned a value. I wrote an `async fn` for the test, put `#[tokio::test]` right above it, and ran `cargo test`. And you know what? It just worked! No fuss, no manual runtime spinning. That was a genuine “aha!” moment for me. Like, where has this been all my (async testing) life?
What It Actually Does, From What I’ve Seen
From my practice, this `#[tokio::test]` macro is basically a neat little wrapper. It takes your `async` test function and handles all the Tokio runtime initialization stuff behind the scenes. So, inside your test, you can use `await` freely, just like you would in your main application code. It sets up a Tokio runtime specifically for that test to run in. This means each test gets its own little isolated async environment, which is pretty handy.
I started using it everywhere. For instance, I had parts of my code that would involve sending a message over a Tokio channel and then waiting for a response or some processing to happen. Before `#[tokio::test]`, trying to test this was a nightmare. I’d have to manually manage the runtime, spawn tasks, and figure out how to wait for things correctly within the test context. But with `#[tokio::test]`, I could just write:
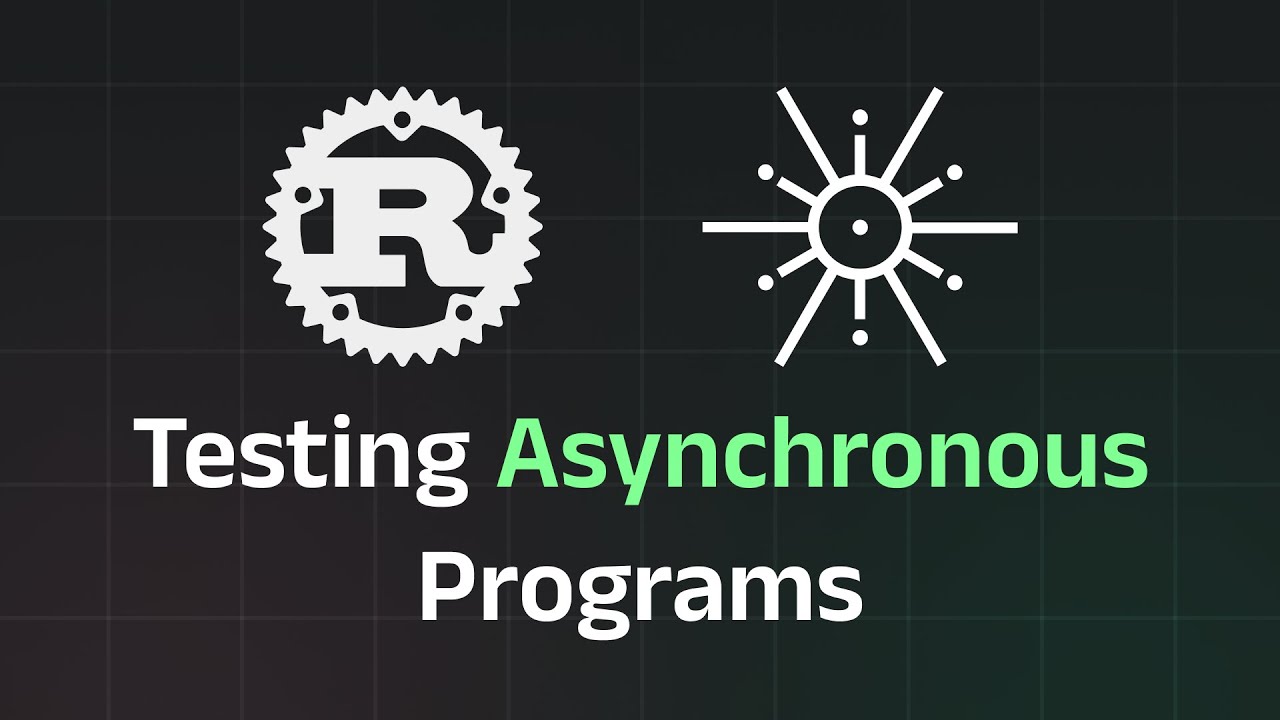
- An async test function.
- Inside, I’d set up my `mpsc::channel`.
- I could `tokio::spawn` a little task to send a message if needed.
- And then, in the main test body, I could `await` the part of my code that was supposed to receive and process that message.
- Finally, assert the outcome.
It made testing these interactive async components so much cleaner. I could simulate timeouts, successful message transfers, all within a test that looked almost as straightforward as a synchronous one.
Things I Genuinely Liked
So, after using it for a while, here’s what stood out to me:
- It’s dead simple. Seriously, just add `#[tokio::test]`. You’re good to go. No complex setup.
- Cleaner tests. My test files are no longer cluttered with boilerplate for setting up a runtime. The focus is purely on the test logic.
- It just works. For 95% of my Tokio-based async testing needs, it does exactly what I expect. It runs my async code.
Any Catches? Well, A Few Minor Things…
Now, it’s not a magic silver bullet for every single complex scenario, but it’s close. The main thing is, well, it’s Tokio’s test macro. If you’re using a different async runtime, this isn’t for you. It ties your tests to the Tokio ecosystem, which is fine if your whole project is already in it, like mine was.
Also, if you have really complex background tasks spawned within your tests that need super careful shutdown logic to prevent flakes, you might still need to put some thought into it. The macro provides the runtime, but if your test spawns tasks that outlive the main test body or don’t get `join`ed properly, you could see some occasional weirdness or warnings about leaked tasks. So, for super intricate stuff, I still had to be mindful of how my spawned tasks were being handled, ensuring they completed or were cancelled before the test function itself exited. But for the most part, for testing individual components or functions, it’s been smooth sailing.
My Takeaway
So, yeah, `#[tokio::test]` has pretty much become a default for me whenever I’m writing tests for Tokio-based code. It took a lot of the pain out of async testing in Rust. Before, I’d kind of dread getting to the testing phase of an async module, but now it feels much more integrated and natural. If you’re deep in Tokio and you’re still manually setting up runtimes for your tests, I’d say give `#[tokio::test]` a serious look. It really did make my development cycle a lot smoother and my tests way more readable. It’s one of those small things that makes a big difference in day-to-day coding.