Alright, so yesterday, I was reading an article about this thing called the “Modric Number.” Never heard of it before, but it sounded kinda cool. The article mentioned how it’s a way to describe positive integers and how you can check if a number is a Modric number or not, so I thought, “Why not give it a shot and try to write some code for it?”
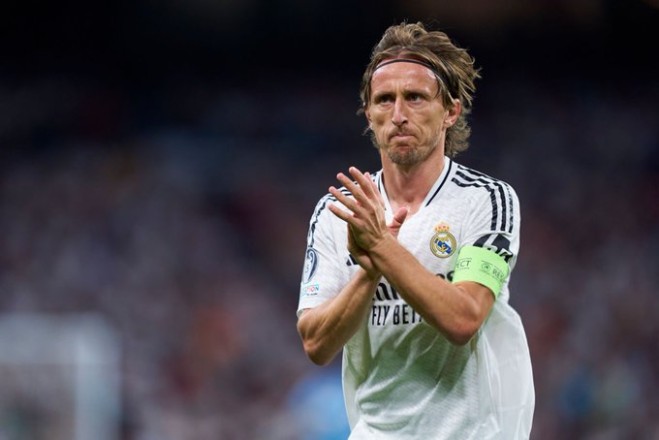
First, I needed to understand what the heck a Modric number actually is. Turns out, it’s a number that can be written as the sum of three cubes in a particular way. You take a positive integer, cube it, and then you need to find two other positive integers that you can also cube and add together to get the same result. Sounds simple enough, right?
Getting Started
I fired up my code editor and started thinking about how to approach this. I figured I’d need a function that takes a number as input and then checks if it meets the criteria. I decided to call it isModricNumber. Nice and straightforward.
- First I need to handle any input that’s not positive, because the definition clearly says positive integer, so I added a check at the start to immediately return false if the number is less than or equal to 0.
- Then, I calculated the cube of the input number. Let’s call this target.
- Next, I started looping through possible values for the first of the two other cubes. I called this number a, and its cube aCubed.
- Inside this loop, I calculated what the remaining value would need to be to reach the target. This would be target minus aCubed. Let’s call this bCubed.
- Now, I needed to check if bCubed is actually a perfect cube. I did this by taking the cube root of bCubed and then checking if cubing that result gets me back to bCubed.
- If it is, then bingo! We’ve found a combination that works, so the function should return true.
- If I go through all possible values of a and don’t find a working combination, then the number isn’t a Modric number, and the function returns false.
Coding It Up
I wrote the code, following the steps I outlined above. It wasn’t too tricky, just a couple of loops and some basic math. I made sure to test it with some simple examples that I could verify by hand. For example, I tried it with 6 (which I knew should work because 6^3 = 216 and 3^3 + 3^3 + 6^3 = 216) and with some numbers I knew wouldn’t work, like 2.
The Result
After a few tweaks and making sure I didn’t mess up any of the calculations, I had a working function! It correctly identified whether a number was a Modric number or not. Pretty neat, huh? I played around with it a bit more, trying out different inputs and seeing what it would spit out. It’s always satisfying to see your code do what it’s supposed to do.
So, that’s my little adventure with Modric numbers. It was a fun way to kill some time and learn something new. Maybe I’ll try to optimize the code later or explore some other interesting number patterns. Who knows? The world of math is full of surprises.
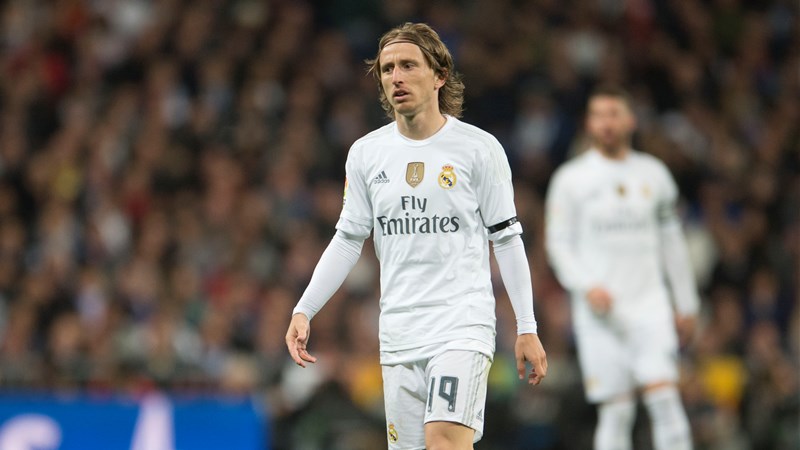