Okay, so I messed around with this “atlantic coast conference coin” thing today, and lemme tell ya, it was a journey.
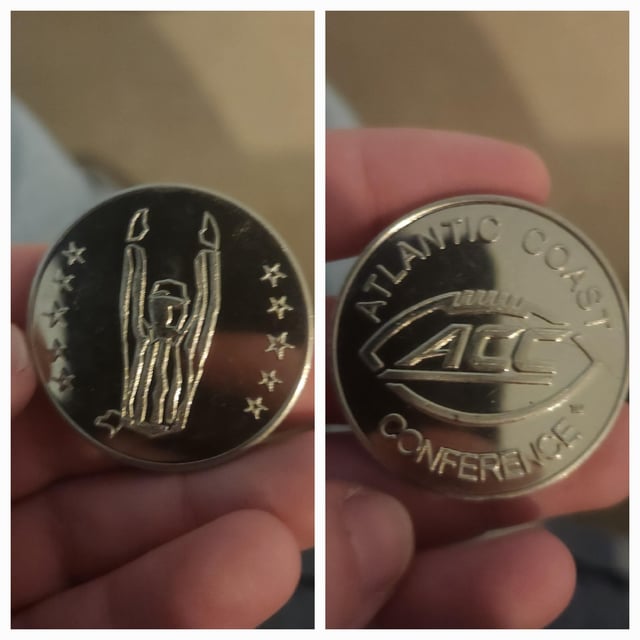
First off, I saw some chatter about it online, people flipping these virtual coins and stuff. Seemed kinda dumb, but I was bored, so I figured, why not?
I started by trying to find an actual “atlantic coast conference coin” – like, a physical coin. Spent a solid hour searching online, but all I found was merch with the ACC logo. Strike one. Seems like it’s just a thing people made up for fun.
Next, I thought, “Alright, if it’s not a real coin, maybe there’s an app or a website?” Nope. More fruitless searching. At this point, I’m thinking, “Okay, people are just making this up as they go.”
So, I decided to embrace the chaos and just make my own virtual coin flip thing. I fired up my Python editor – yeah, I’m a bit of a nerd – and started banging out some code.
Here’s what I did:
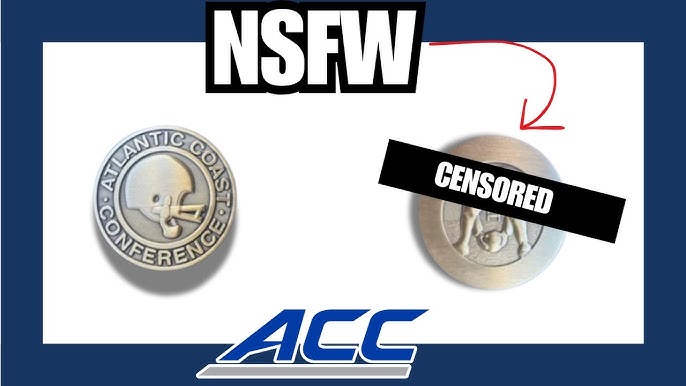
- Imported the
random
module. Super important for, you know, random stuff. - Defined a function called
flip_coin()
. This is where the magic happens. - Inside the function, I used
*(['Heads', 'Tails'])
to randomly pick either “Heads” or “Tails”. - Then, I just printed the result to the console. Simple as that.
python
import random
def flip_coin():
result = *([‘Heads’, ‘Tails’])
print(result)
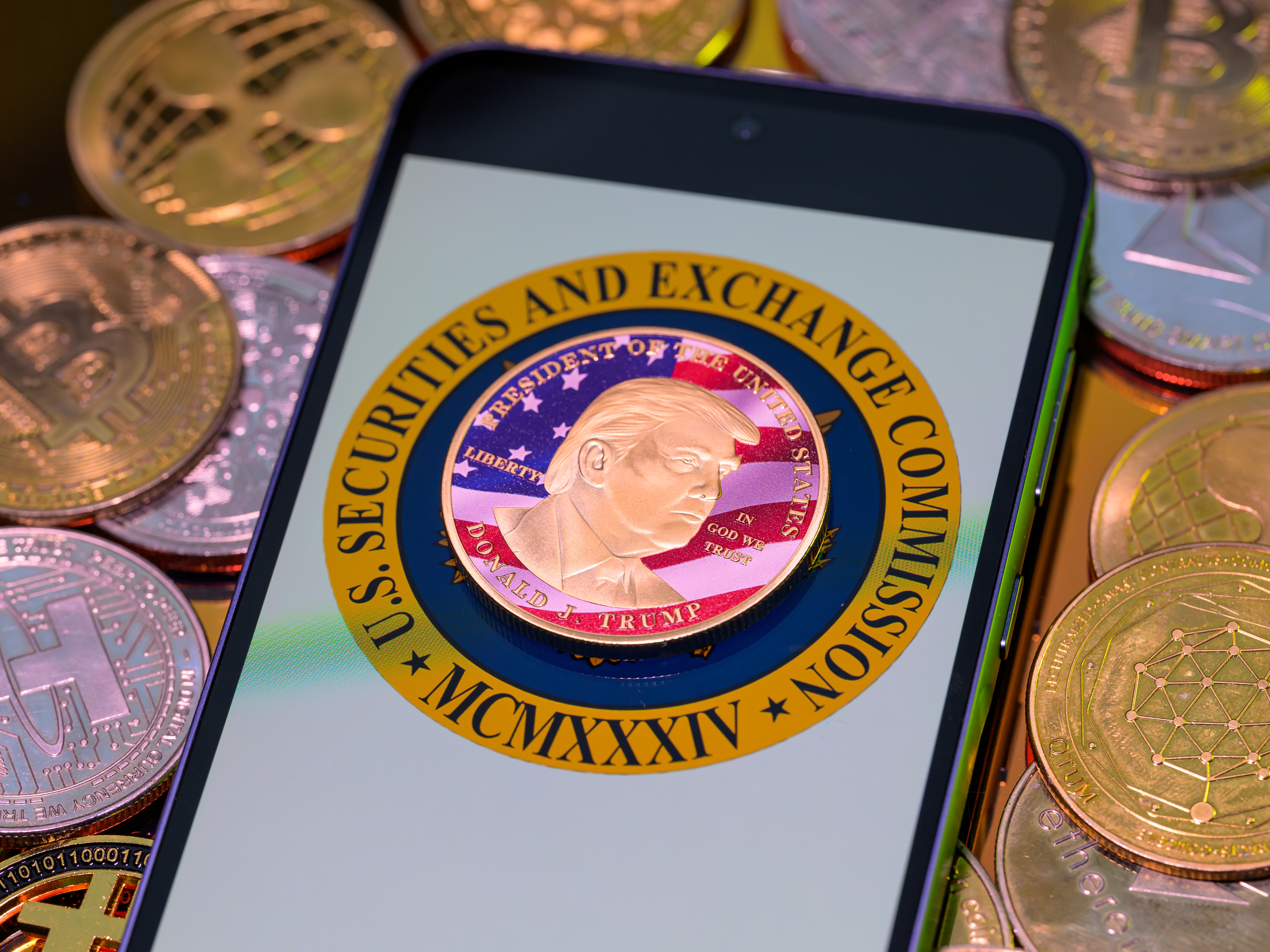
flip_coin()
It ain’t pretty, but it works. Now I can run the script and get a random “Heads” or “Tails” every time.
But that wasn’t enough. I wanted to make it ACC-themed, somehow. So, I decided to get really dumb with it.
I changed the to use ACC teams instead of “Heads” and “Tails”. Something like:

python
import random
def acc_flip():
teams = [‘Clemson’, ‘FSU’, ‘Miami’, ‘UNC’, ‘Duke’, ‘NC State’, ‘Pitt’, ‘Wake Forest’, ‘Louisville’, ‘Virginia Tech’, ‘Georgia Tech’, ‘Boston College’, ‘Syracuse’, ‘Virginia’, ‘California’, ‘Stanford’, ‘SMU’]
result = *(teams)
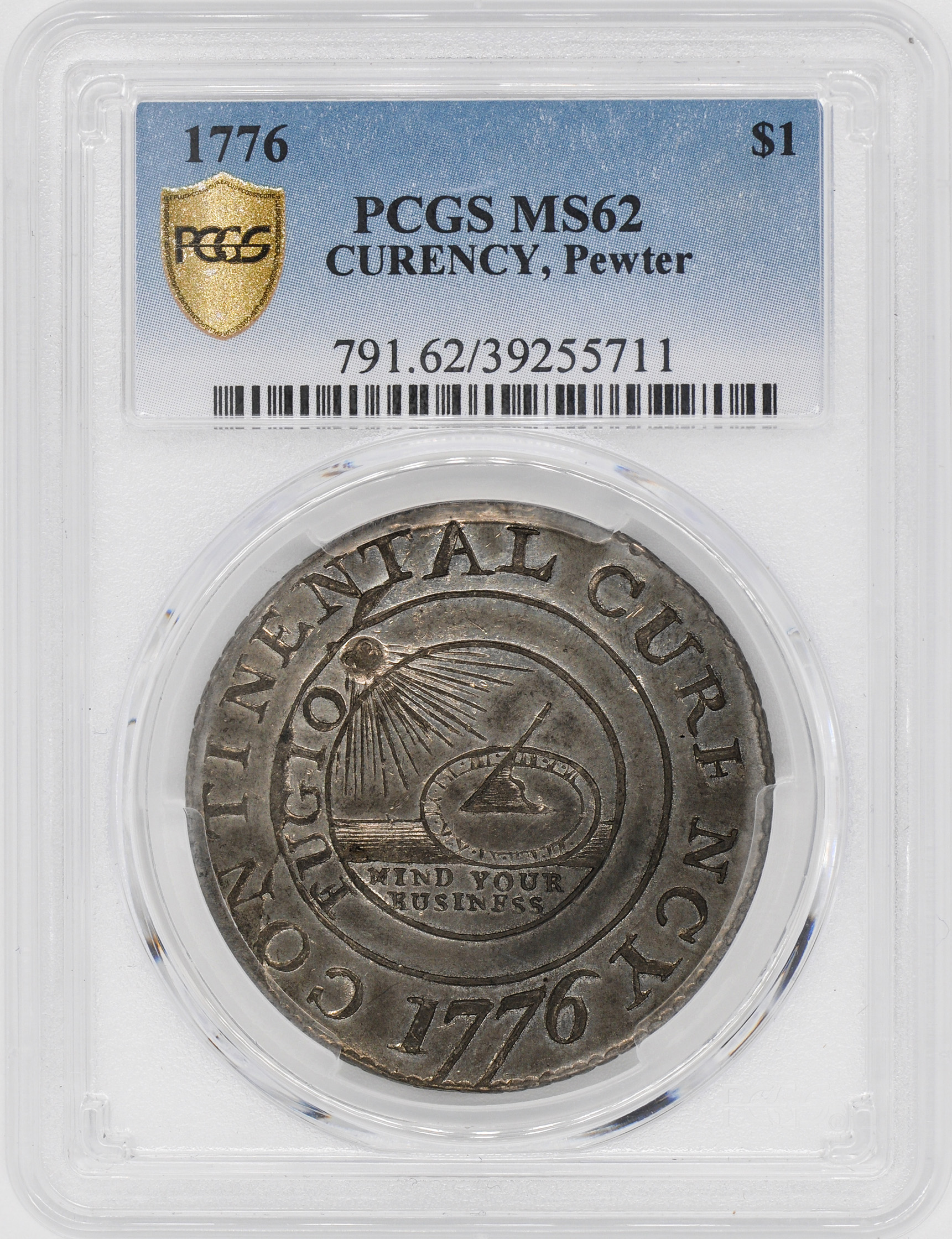
print(result)
acc_flip()
Now, when I run the script, it spits out a random ACC team. What does it mean? Absolutely nothing. But hey, it’s “atlantic coast conference coin”-ish, right?
Finally, just for kicks, I hooked it up to a simple web page using Flask. Basically, a button that, when clicked, runs the Python script and displays the result on the page. Nothing fancy, but it was fun to do.
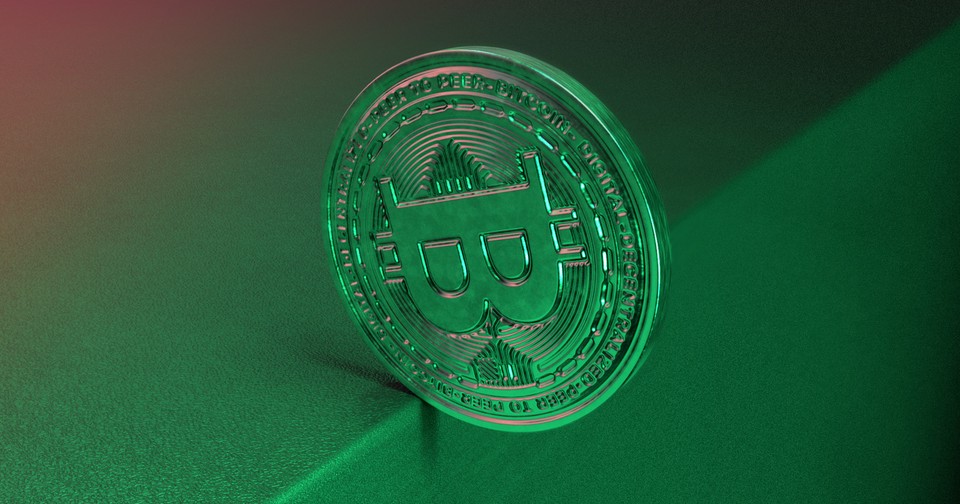
So, yeah, that’s my “atlantic coast conference coin” adventure. Turns out, it’s not a real thing, but I made my own janky version. Don’t ask me why, I have no idea. Maybe I just needed a distraction.
The moral of the story? Sometimes, the best things are the ones you make up yourself. And Python is awesome.