Okay, so I wanted to mess around with creating some custom paint effects in Ruby, because why not? I’d seen some cool procedural art things online and thought, “I bet I can do something like that.”
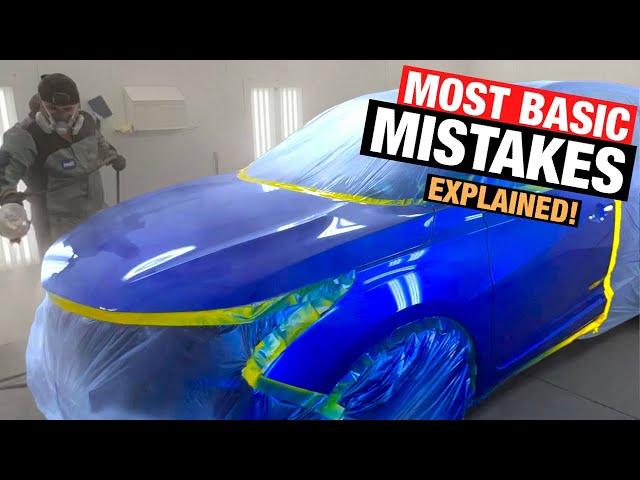
I started by just getting a basic window up and running. I used the ‘gosu’ gem. I remember needing to install that first. It went like this:
ruby
gem install gosu
I slammed that into the terminal. Easy peasy.
Setting up the Basic Window
Then, I created a new Ruby file, I called it ‘rb_*’, classic. I needed the basic Gosu stuff to make a window appear.
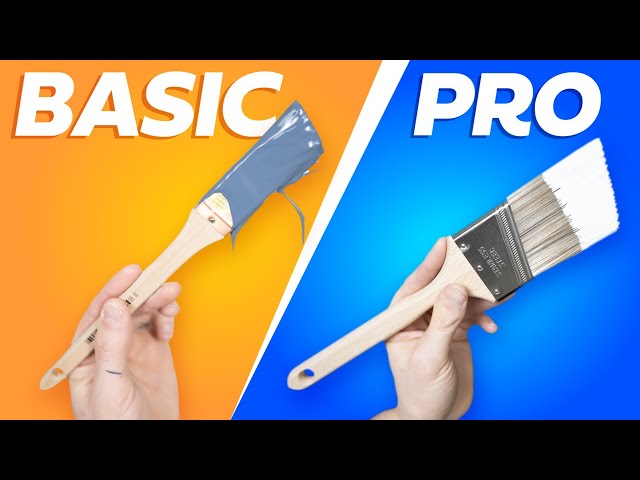
Here’s what that looked like.
ruby
require ‘gosu’
class MyWindow < Gosu::Window
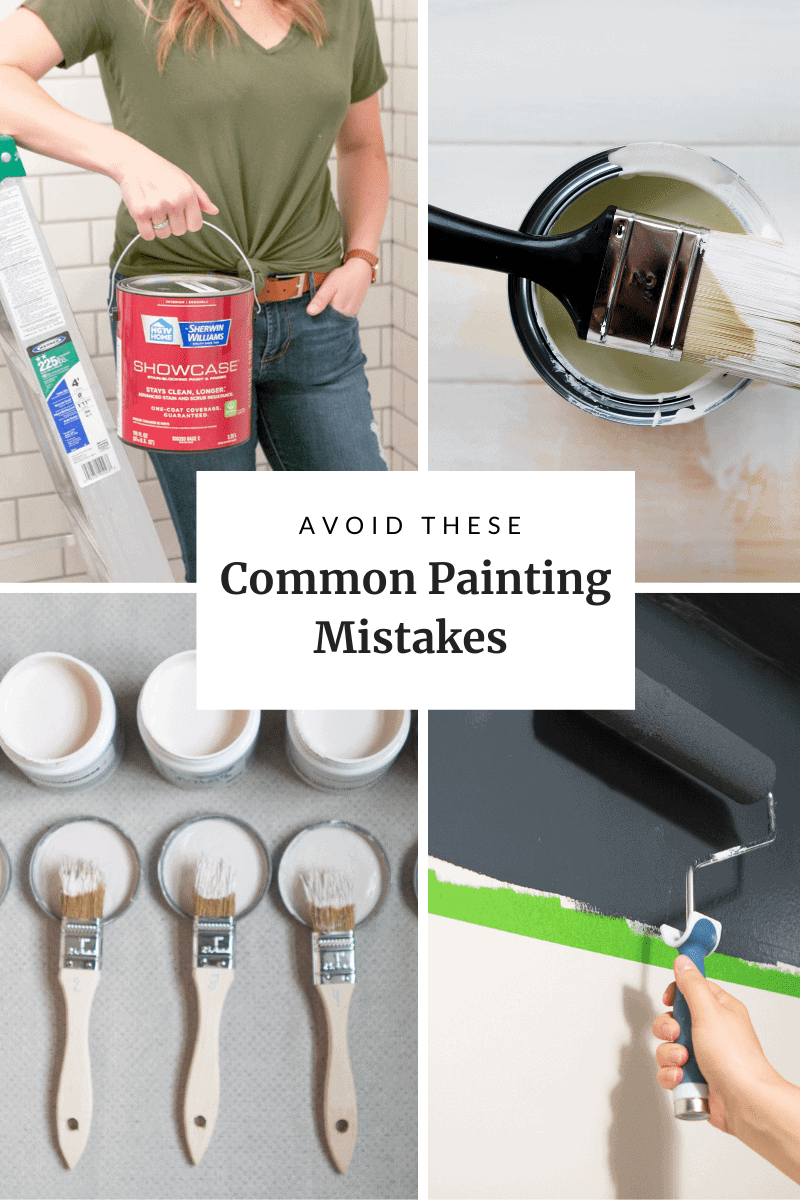
def initialize
super 640, 480
* = “My Awesome Paint App”
end
def draw
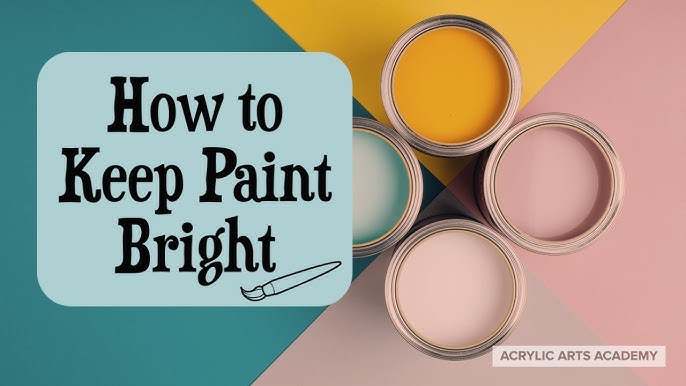
# We’ll get to the drawing part…
end
end
window = *
I made a class, MyWindow, inherited from Gosu::Window. The initialize method sets the width and height, and the window caption. The draw method… well, that’s where the painting magic will eventually happen. Finally, I created an instance of MyWindow and called show to make it appear.
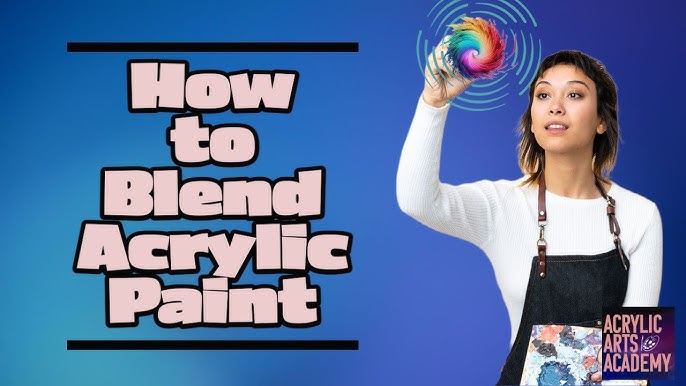
I ran it:
bash
ruby rb_*
Boom! A blank window. Progress!
Adding Mouse Tracking
Next, I wanted to know where my mouse was. I added these methods to my MyWindow class:
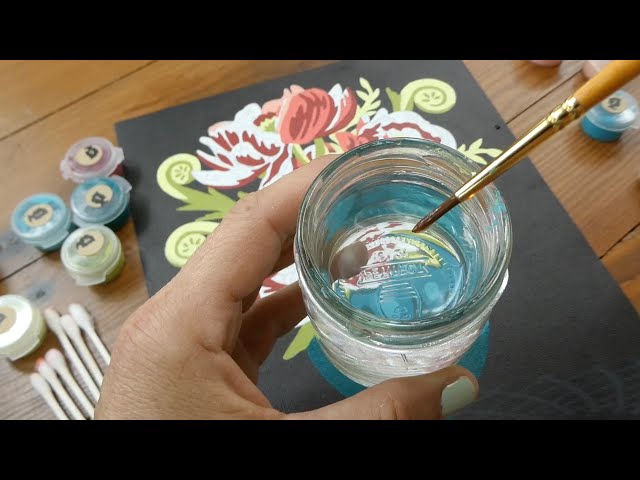
ruby
def needs_cursor?
true
end
def update
#nothing yet
end
The needs_cursor? method, when returning true, just tells Gosu to show the mouse cursor. which makes the mouse visible.
Then, I added the update.
Simple Circle Drawing
Now for the fun part. Drawing! I wanted to start simple, just drawing a circle wherever the mouse is. I added this inside the draw method:
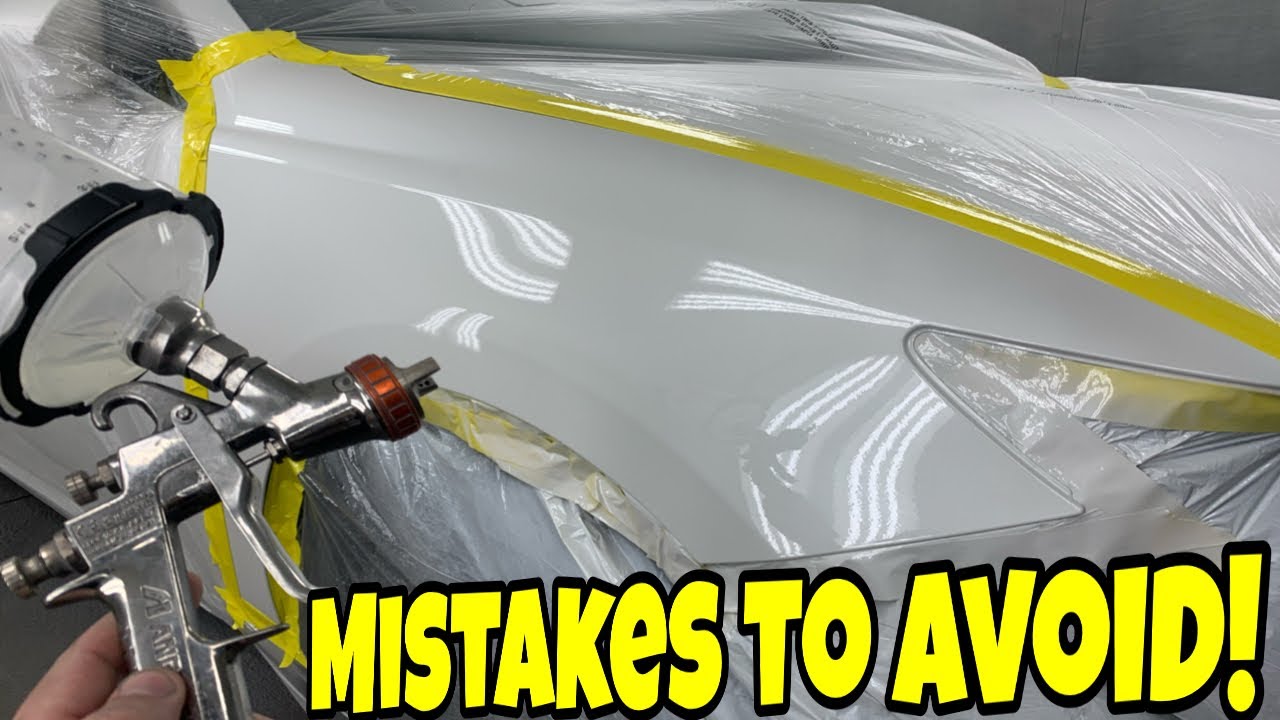
ruby
draw_quad(
mouse_x – 5, mouse_y – 5, Gosu::Color::WHITE,
mouse_x + 5, mouse_y – 5, Gosu::Color::WHITE,
mouse_x – 5, mouse_y + 5, Gosu::Color::WHITE,
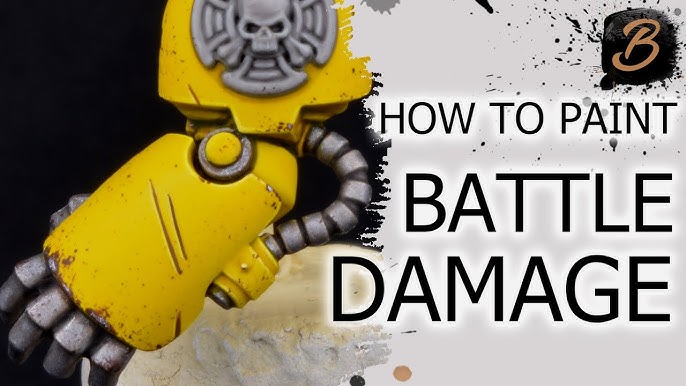
mouse_x + 5, mouse_y + 5, Gosu::Color::WHITE,
0
I use the draw_quad method, which is a quick way to draw a rectangle.
I set the color to white for now and used the mouse_x and mouse_y values.
I ran it again, and hey, I had a little white square following my mouse!
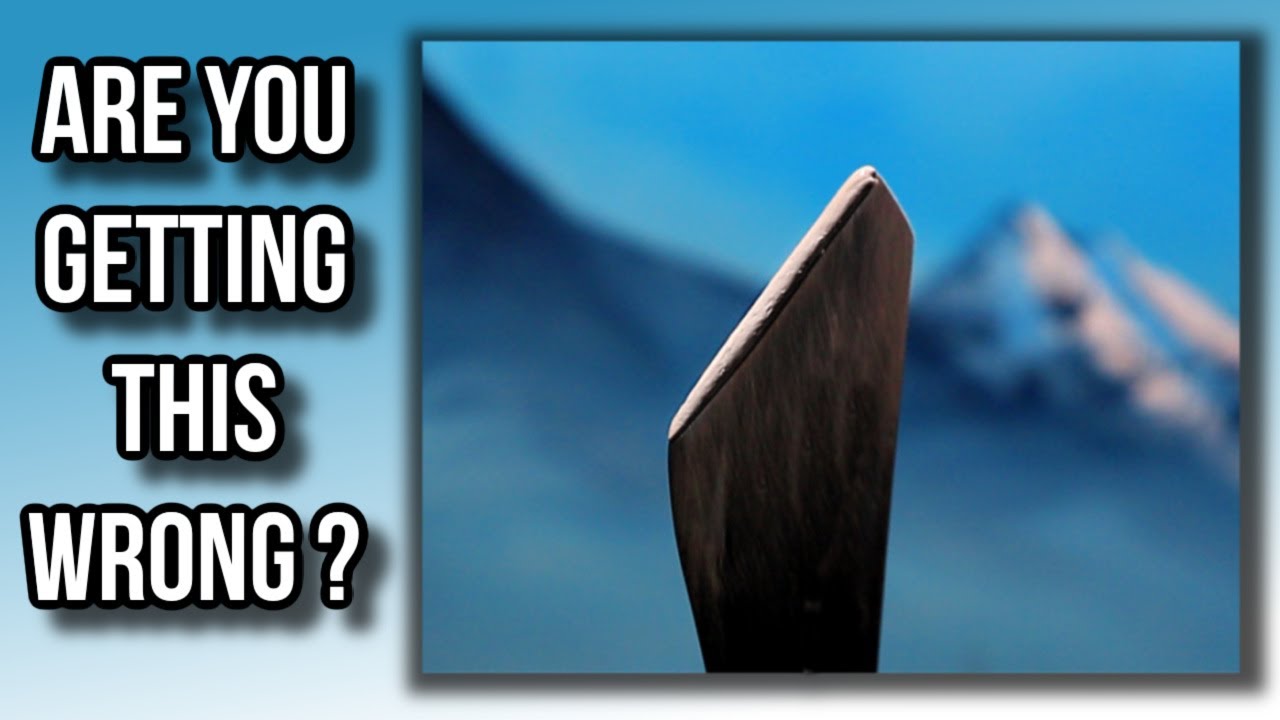
Leaving a Trail
This was cool, but I wanted a trail. So, I needed to remember where the mouse had been. I added an array to store the positions, added this in the initialize.
ruby
@positions = []
Then in the update method.
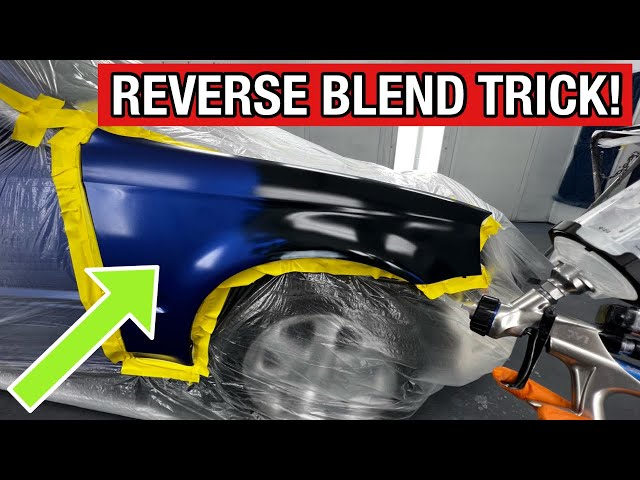
ruby
@positions << [mouse_x, mouse_y]
@* if @* > 50 # Keep the trail limited
and finally changed the draw method to this.
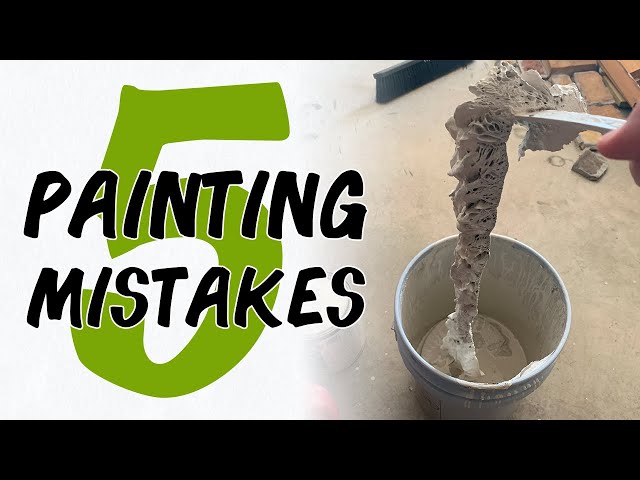
ruby
@* do pos
draw_quad(
pos[0] – 5, pos[1] – 5, Gosu::Color::WHITE,
pos[0] + 5, pos[1] – 5, Gosu::Color::WHITE,
pos[0] – 5, pos[1] + 5, Gosu::Color::WHITE,
pos[0] + 5, pos[1] + 5, Gosu::Color::WHITE,
0
end
I’m storing the mouse positions in the @positions array. And, importantly, I’m limiting the array’s size to 50 elements, so the trail doesn’t get infinitely long. Now, in draw, I’m iterating through @positions and drawing a square for each past position.
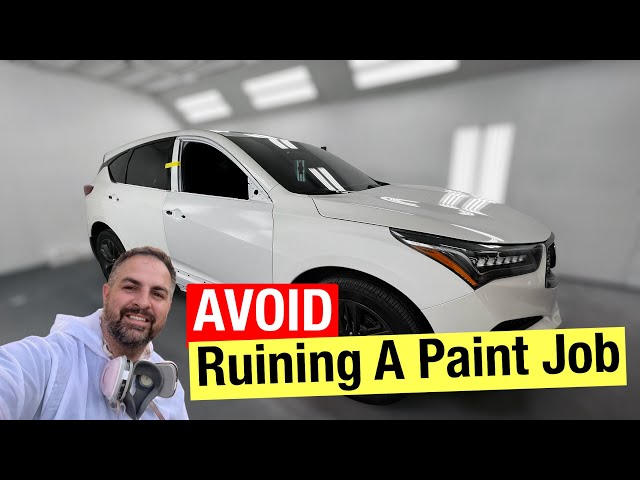
Ran it. Awesome! A white trail! It’s basic, but it’s my basic trail.
Adding Some Color
White is boring. I wanted color! I decided to make the color change based on the mouse’s x-coordinate. I changed draw to this:
ruby
@* do pos
color = Gosu::*(0xff_000000)
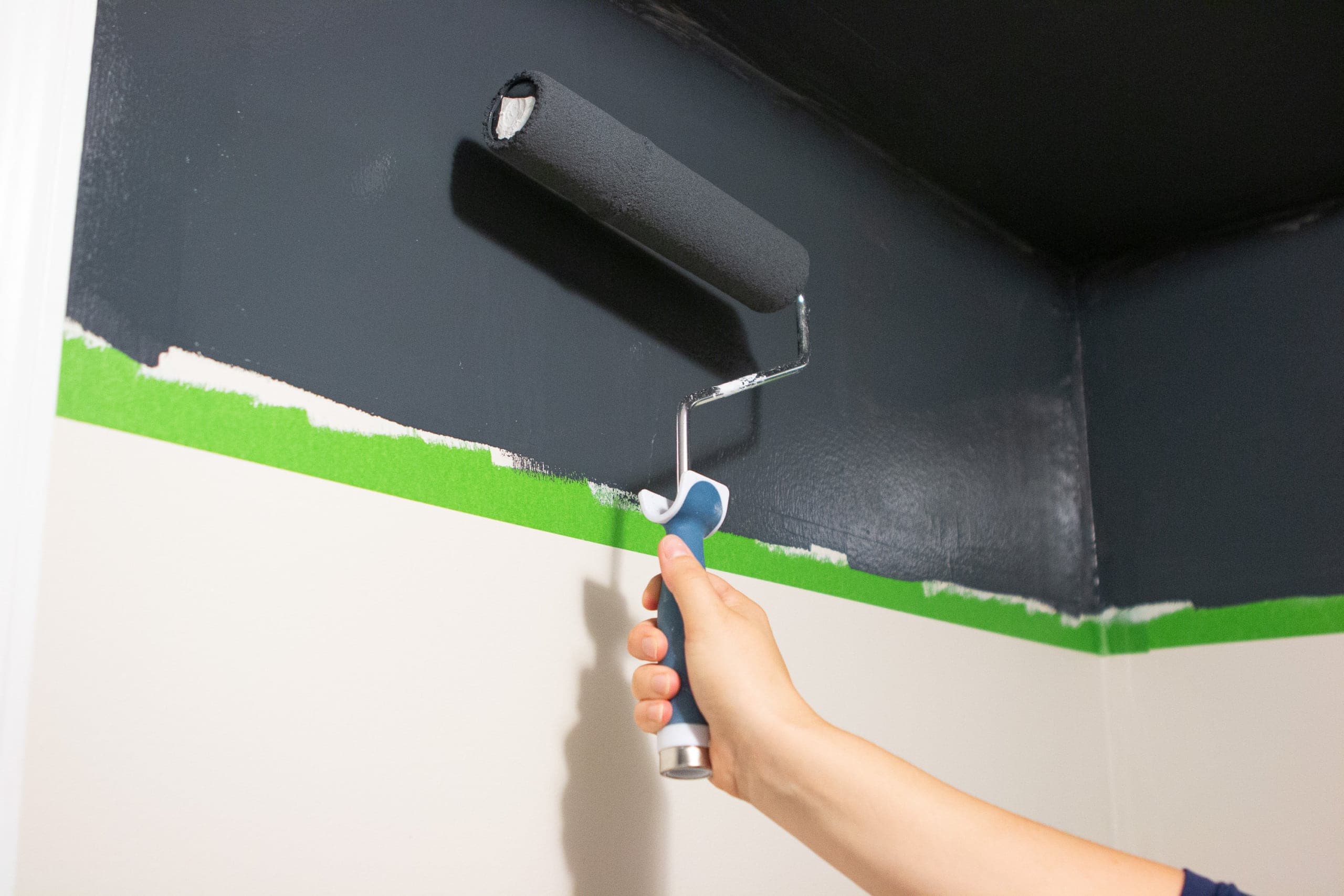
* = (pos[0] % 255)
* = (pos[0] % 255)
* = (pos[1] % 255)
draw_quad(
pos[0] – 5, pos[1] – 5, color,
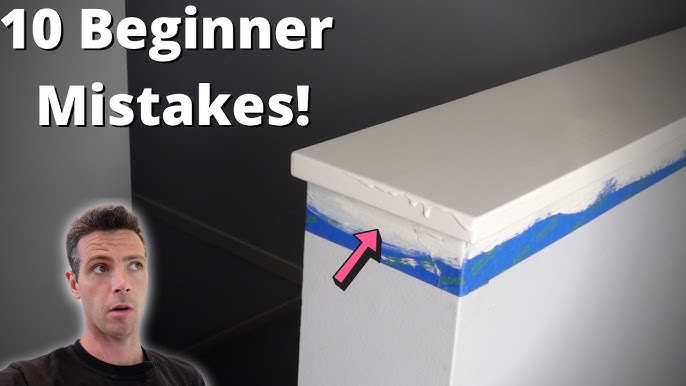
pos[0] + 5, pos[1] – 5, color,
pos[0] – 5, pos[1] + 5, color,
pos[0] + 5, pos[1] + 5, color,
0
end
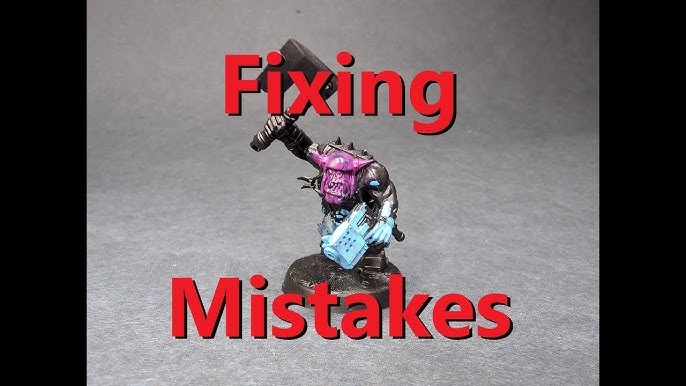
And added color.
I created a Gosu::Color object. Then, I set its red and green components based on the x-position and blue based on y. I used the modulo operator (%) to keep the values within the 0-255 range.
Now I had a colorful trail! It felt way more alive.
This is where I stopped for the day. It’s a simple start, but it’s a good foundation. I can add more complex shapes, different color effects, maybe even some user input to change parameters. It’s pretty cool to see how a few lines of code can start to create something visual and interactive. I’m excited to keep playing with this!